Arrays are a fundamental concept in computer programming that allow developers to store and manipulate collections of data. In simple terms, an array is a data structure that can hold multiple values of the same data type in a single variable. This makes it easier to work with large sets of data and perform operations on them efficiently.
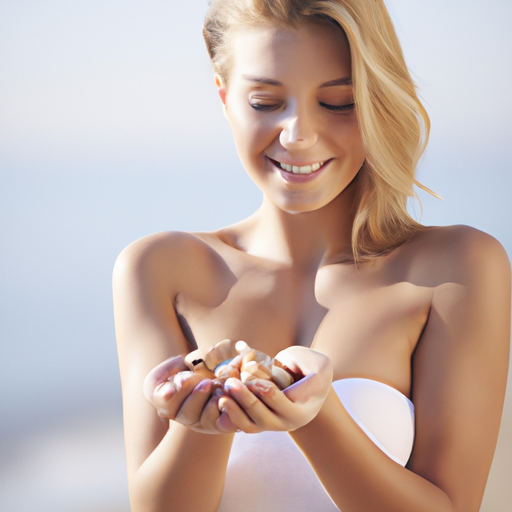
What is an Array?
An array is a collection of elements, each identified by an index or key. The elements in an array are typically of the same data type, such as integers, strings, or objects. Arrays are used to store and organize data in a structured way, making it easier to access and manipulate individual elements.
Arrays are commonly used in programming languages like C, Java, Python, and JavaScript. In most languages, arrays are zero-indexed, meaning that the first element in the array is at index 0, the second element is at index 1, and so on. This allows developers to access elements in an array by their position in the collection.
How Do Arrays Work?
Arrays are implemented as contiguous blocks of memory in computer memory. When you create an array in your code, the programming language allocates a specific amount of memory to store the elements of the array. Each element is stored at a specific memory address, which can be accessed using the index of the element.
For example, if you have an array of integers in C with five elements, the memory layout might look like this:
Index 0: 10 Index 1: 20 Index 2: 30 Index 3: 40 Index 4: 50
To access the element at index 2 in the array, you would use the syntax array[2], which would return the value 30. This allows you to easily retrieve and modify individual elements in the array using their index.
Types of Arrays
There are several types of arrays that are commonly used in programming, each with its own characteristics and use cases. Some of the most common types of arrays include:
1. One-dimensional arrays: These are the simplest form of arrays, where elements are arranged in a single row or column. One-dimensional arrays are used to store a list of values that can be accessed using a single index.
2. Multi-dimensional arrays: These arrays have more than one dimension, such as rows and columns in a table. Multi-dimensional arrays are used to store complex data structures, such as matrices or tables.
3. Dynamic arrays: These arrays can grow or shrink in size dynamically at runtime. Dynamic arrays are useful when the size of the array is not known in advance or needs to change frequently.
4. Jagged arrays: These arrays are arrays of arrays, where each element in the array is itself an array. Jagged arrays are used to store data structures of varying lengths, such as lists of lists.
Common Operations on Arrays
Arrays support a variety of operations that allow developers to manipulate and work with the data stored in the array. Some of the most common operations on arrays include:
1. Accessing elements: You can access individual elements in an array using their index. This allows you to retrieve and modify specific values in the array.
2. Adding elements: You can add new elements to an array by assigning a value to a specific index or using functions like push() in languages like JavaScript.
3. Removing elements: You can remove elements from an array by setting the value at a specific index to null or using functions like pop() or splice() to remove elements from the array.
4. Searching for elements: You can search for specific elements in an array using functions like indexOf() or find() in languages like JavaScript.
5. Sorting elements: You can sort the elements in an array in ascending or descending order using functions like sort() or reverse().
Best Practices for Using Arrays
When working with arrays in your code, there are some best practices that you should follow to ensure efficient and maintainable code. Some of these best practices include:
1. Use meaningful variable names: When declaring arrays, use descriptive variable names that indicate the purpose or contents of the array. This makes your code easier to understand and maintain.
2. Avoid magic numbers: Instead of hardcoding array sizes or indexes in your code, use constants or variables to represent these values. This makes your code more flexible and easier to update in the future.
3. Initialize arrays properly: Always initialize arrays with default values or populate them with data before using them in your code. This helps prevent errors and unexpected behavior when working with arrays.
4. Use built-in array functions: Many programming languages provide built-in functions for working with arrays, such as sorting, filtering, and mapping. Use these functions instead of writing custom code to perform common operations on arrays.
5. Handle errors and edge cases: When working with arrays, be mindful of edge cases like empty arrays, out-of-bounds indexes, or null values. Always check for these cases and handle them gracefully in your code.
In conclusion, arrays are a powerful and versatile data structure that is essential for working with collections of data in computer programming. By understanding how arrays work, the different types of arrays available, common operations that can be performed on arrays, and best practices for using arrays in your code, you can become a more effective and efficient programmer. Arrays are a fundamental concept that every developer should master to write clean, efficient, and maintainable code.